Building a PDF in iOS
Storyboards in Xcode are a great way to build PDFs. But it can be challenging to make PDFs with high resolution without knowing the following trick.
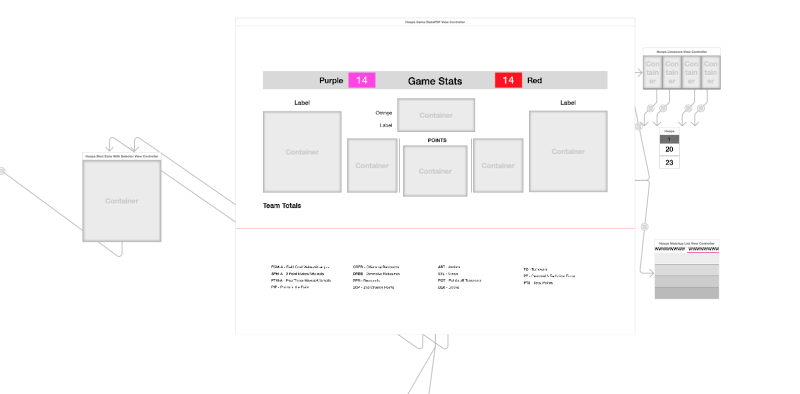
Scaling the PDF
By default, the text resolution for a printed PDF generated by a Storyboard will be mediocre. To make the text much crisper, I recommend the following:
- Double or quadruple all of the measurements on the design spec.
- Build the Storyboard to those specifications
- Before rendering the PDF, shrink all of the elements by 2-4 times.
In the following code block, I am halving the measurements of the view. Halving the measurements quadruples the DpI (Dots per Inch). We are doubling the number of dots in each dimension, which results in a fourfold increase in DpI.
//First PDF Page
UIGraphicsBeginPDFPage()
CGContextSaveGState(pdfContext)
CGContextConcatCTM(pdfContext, CGAffineTransformMakeScale(0.5, 0.5))
let firstPagePdfStoryboard = UIStoryboard(name: "GameStatsPDFCoverPage", bundle: nil)
let firstPagePdfVC = firstPagePdfStoryboard.instantiateViewControllerWithIdentifier("GameStatsPDFCover")
firstPagePdfVC.view.layer.shouldRasterize = false
firstPagePdfVC.view.layer.renderInContext(pdfContext)
CGContextRestoreGState(pdfContext)
At GameChanger we experienced this problem. We were building a rich PDF for basketball coaches to share with players, fans, and the press. By scaling up and then scaling down all the view elements, we were able to build a very high quality PDF. Here it is: